I was very pleased with the code of my MVC application, but the presentation was so 1990's. With all of this new technology I thought the presentation deserved something snappy. That is when I found Flexigrid.
As I mentioned in my last blog entry, I started to use jQuery. To my joy, Flexigrid is a jQuery plugin! Flexigrid uses jQuery to asynchronously populate the contents of the grid using either XML or JSON input.
The following is an example of what the grid looks like. It contains features to sort, page, search, move columns, resize, etc…
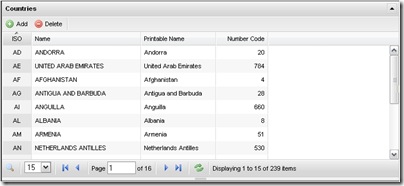
The User Interface portion was pretty straightforward to put together. You just need to define your columns, the data source, and some additional parameters (such as: search terms, size, etc…).
I wanted to use this opportunity to try out some new features of .NET 3.5, so I wanted to incorporate LINQ and JSON serialization.
To do this, I needed to setup some classes that the Flexigrid will recognize once serialized. Here is what I came up with.
1: public class FlexigridViewData
2: {
3: public int page;
4: public int total;
5: public List<FlexigridRow> rows = new List<FlexigridRow>();
6: }
7:
8: public class FlexigridRow
9: {
10: public long id;
11: public List<string> cell;
12: }
1: public void Page_Load()
2: {
3: Response.Clear();
4: Response.ContentType = "text/x-json";
5: Response.Write(GetPagedContent());
6: Response.Flush();
7: Response.End();
8: }
9:
10: private string GetPagedContent()
11: {
12: var pageIndex = Convert.ToInt32(Request.Params["page"]);
13: var itemsPerPage = Convert.ToInt32(Request.Params["rp"]);
14: var sortName = Request.Params["sortname"];
15: var sortOrder = Request.Params["sortorder"];
16: var query = Request.Params["query"];
17:
18: IEnumerable<Contact> pagedContacts;
19: if (string.IsNullOrEmpty(query))
20: {
21: pagedContacts = sortOrder.Equals("asc") ?
22: Contacts.OrderBy(contact => contact.GetPropertyValue<IComparable>(sortName)) :
23: Contacts.OrderByDescending(contact => contact.GetPropertyValue<IComparable>(sortName));
24: }
25: else
26: {
27: Func<Contact, bool> whereClause = (contact => contact.GetPropertyValue<string>(sortName).Contains(query));
28: pagedContacts = sortOrder.Equals("asc", StringComparison.CurrentCultureIgnoreCase) ?
29: Contacts.Where(whereClause).OrderByDescending(contact => contact.GetPropertyValue<IComparable>(sortName)) :
30: Contacts.Where(whereClause).OrderBy(contact => contact.GetPropertyValue<IComparable>(sortName));
31: }
32: int count = pagedContacts.Count();
33: pagedContacts = pagedContacts.Skip((pageIndex - 1) * itemsPerPage).Take(itemsPerPage);
34:
35: const string imageLinkFormat = @"<a href=""{0}""><img src=""{1}"" border=""0"" /></a>";
36: const string imageFormat = @"<img src=""{0}"" border=""0"" />";
37: var flexigrid = new FlexigridViewData {page = pageIndex, total = count};
38: foreach (var contact in pagedContacts)
39: {
40: flexigrid.rows.Add(new FlexigridRow
41: {
42: id = contact.ID,
43: cell = new List<string>
44: {
45: string.Format(imageLinkFormat, ResolveUrl("~/Contact.mvc/Detail/" + contact.ID),
46: ResolveUrl("~/Images/Detail.gif")),
47: contact.ID.ToString(),
48: contact.FirstName,
49: contact.LastName,
50: contact.DateOfBirth.ToShortDateString(),
51: }
52: });
53: }
54:
55: return flexigrid.ToJson();
56: }
1: public static class JsonHelper
2: {
3: public static string ToJson(this object obj)
4: {
5: var serializer = new JavaScriptSerializer();
6:
7: return serializer.Serialize( obj );
8: }
9:
10: public static string ToJson(this object obj, int recursionDepth)
11: {
12: var serializer = new JavaScriptSerializer();
13:
14: serializer.RecursionLimit = recursionDepth;
15:
16: return serializer.Serialize( obj );
17: }
18: }
1: public static T GetPropertyValue<T>(this object component, string propertyName)
2: {
3: return (T) TypeDescriptor.GetProperties(component)[propertyName].GetValue(component);
4: }
I would prefer if the developer of the product had a better system of tracking bugs and maintaining a forum, but in the meantime what is setup is sufficient.
Hi,
ReplyDeleteWhat were the javascript fixes you had to make?
Thanks for the example by the way.
I think the error is with json return, something about a comma ,
ReplyDeleteread the forum on codigniter
Hi Elijah
ReplyDeleteI will definitively check this out, and maybe even use it in my jQuery MVC plugins. I think the grid would be useful when scaffolding JSON result, and maybe add some editing features as well. Just got back from vacation, so I’ve plenty of things to do, but I hopefully get some spare time soon! Sorry for the short a not so insightful comment, but I will get back to you when I start looking at this:)
Good post!
Great, I am actually about to do some work with a grid view and this'll fit in nicely.
ReplyDelete