Thursday, July 31, 2008
Design Pattern Riddle #14
they were mismatched and unable to commune.
Then along came a extraordinary pattern,
that allowed them to sing the same tune.
a. Adapter
~/riddle by me
Wednesday, July 30, 2008
Design Pattern Riddle #13
The kinds that have a common subject.
There is no need to provide specific details,
When using this conceptual mill project.
a. Abstract Factory
~/riddle by me
Tuesday, July 29, 2008
Design Pattern Riddle #12
You don't have to know how their made.
I give a way for each object to shine,
As they walk down the object parade.
a. Iterator
~/riddle by me
Monday, July 28, 2008
Design Pattern Riddle #11
But I'm not the kind who likes to crow.
I gladly give power to those that inherit;
I'm the first to tell the object, "hello!"
a. Factory Method
~/riddle by me
Friday, July 25, 2008
Design Pattern Riddle #10
behind the scenes I resemble a deep rooted plant.
I contain a family of parents and children,
but no where in me can you find an aunt.
a. Composite
~/riddle by me
Thursday, July 24, 2008
Design Pattern Riddle #9
I am highly abstract, but generically planned.
A superclass can localize your common behavior,
but there is plenty of room for you to command.
a. Template Method
~/riddle by me
Wednesday, July 23, 2008
jQuery Flexigrid Using C# 3.0 (.NET 3.5) & LINQ
I was very pleased with the code of my MVC application, but the presentation was so 1990's. With all of this new technology I thought the presentation deserved something snappy. That is when I found Flexigrid.
As I mentioned in my last blog entry, I started to use jQuery. To my joy, Flexigrid is a jQuery plugin! Flexigrid uses jQuery to asynchronously populate the contents of the grid using either XML or JSON input.
The following is an example of what the grid looks like. It contains features to sort, page, search, move columns, resize, etc…
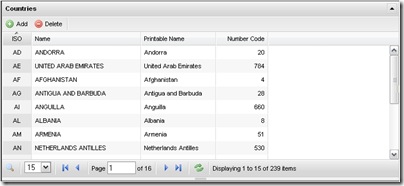
The User Interface portion was pretty straightforward to put together. You just need to define your columns, the data source, and some additional parameters (such as: search terms, size, etc…).
I wanted to use this opportunity to try out some new features of .NET 3.5, so I wanted to incorporate LINQ and JSON serialization.
To do this, I needed to setup some classes that the Flexigrid will recognize once serialized. Here is what I came up with.
1: public class FlexigridViewData
2: {
3: public int page;
4: public int total;
5: public List<FlexigridRow> rows = new List<FlexigridRow>();
6: }
7:
8: public class FlexigridRow
9: {
10: public long id;
11: public List<string> cell;
12: }
1: public void Page_Load()
2: {
3: Response.Clear();
4: Response.ContentType = "text/x-json";
5: Response.Write(GetPagedContent());
6: Response.Flush();
7: Response.End();
8: }
9:
10: private string GetPagedContent()
11: {
12: var pageIndex = Convert.ToInt32(Request.Params["page"]);
13: var itemsPerPage = Convert.ToInt32(Request.Params["rp"]);
14: var sortName = Request.Params["sortname"];
15: var sortOrder = Request.Params["sortorder"];
16: var query = Request.Params["query"];
17:
18: IEnumerable<Contact> pagedContacts;
19: if (string.IsNullOrEmpty(query))
20: {
21: pagedContacts = sortOrder.Equals("asc") ?
22: Contacts.OrderBy(contact => contact.GetPropertyValue<IComparable>(sortName)) :
23: Contacts.OrderByDescending(contact => contact.GetPropertyValue<IComparable>(sortName));
24: }
25: else
26: {
27: Func<Contact, bool> whereClause = (contact => contact.GetPropertyValue<string>(sortName).Contains(query));
28: pagedContacts = sortOrder.Equals("asc", StringComparison.CurrentCultureIgnoreCase) ?
29: Contacts.Where(whereClause).OrderByDescending(contact => contact.GetPropertyValue<IComparable>(sortName)) :
30: Contacts.Where(whereClause).OrderBy(contact => contact.GetPropertyValue<IComparable>(sortName));
31: }
32: int count = pagedContacts.Count();
33: pagedContacts = pagedContacts.Skip((pageIndex - 1) * itemsPerPage).Take(itemsPerPage);
34:
35: const string imageLinkFormat = @"<a href=""{0}""><img src=""{1}"" border=""0"" /></a>";
36: const string imageFormat = @"<img src=""{0}"" border=""0"" />";
37: var flexigrid = new FlexigridViewData {page = pageIndex, total = count};
38: foreach (var contact in pagedContacts)
39: {
40: flexigrid.rows.Add(new FlexigridRow
41: {
42: id = contact.ID,
43: cell = new List<string>
44: {
45: string.Format(imageLinkFormat, ResolveUrl("~/Contact.mvc/Detail/" + contact.ID),
46: ResolveUrl("~/Images/Detail.gif")),
47: contact.ID.ToString(),
48: contact.FirstName,
49: contact.LastName,
50: contact.DateOfBirth.ToShortDateString(),
51: }
52: });
53: }
54:
55: return flexigrid.ToJson();
56: }
1: public static class JsonHelper
2: {
3: public static string ToJson(this object obj)
4: {
5: var serializer = new JavaScriptSerializer();
6:
7: return serializer.Serialize( obj );
8: }
9:
10: public static string ToJson(this object obj, int recursionDepth)
11: {
12: var serializer = new JavaScriptSerializer();
13:
14: serializer.RecursionLimit = recursionDepth;
15:
16: return serializer.Serialize( obj );
17: }
18: }
1: public static T GetPropertyValue<T>(this object component, string propertyName)
2: {
3: return (T) TypeDescriptor.GetProperties(component)[propertyName].GetValue(component);
4: }
I would prefer if the developer of the product had a better system of tracking bugs and maintaining a forum, but in the meantime what is setup is sufficient.
Design Pattern Riddle #8
I can be your design of choice.
I can organize your requests
or back them out, it's your choice.
Each object has it's parameters
That tells them how to act.
I am made of generic components;
Each one defines their internal pact.
a. Command
~/riddle by me
Tuesday, July 22, 2008
Design Pattern Riddle #7
a window dressing concealing something immense.
I offer an unified perspective to numerous systems;
I excel at making complex things make sense.
a. Façade
~/riddle by me
Monday, July 21, 2008
jQuery
I have recently started using jQuery and I’ve been quite impressed. I found integrating jQuery most useful while I developed my first ASP.NET MVC Preview 3 application.
jQuery was able to simplify some of the things I would have had to write nasty looking View code otherwise.
For example, I wanted to alternate every other row in a table with a different color. To do this in the View I initially created a counter variable and did a mod 2 to apply the CSS class. Once I integrated jQuery I was able to remove that messy code throughout my entire View and just replace it with…
1: <script type="text/javascript">
2: $(document).ready(function(){
3: $("#tblList tr:odd").addClass("alternatingRow");
4: $("#tblList tr:even").addClass("row");
5: });
6: </script>
After I got a little familiar with jQuery I branched out and used it to create an accordion navigation menu with little effort.
1: $("#menu li div.subMenuItems").hide();
2: $("#menu li div.parentMenuItem").click(function()
3: {
4: $("#menu li div.subMenuItems:visible").slideUp("slow");
5: $(this).next().slideDown("slow");
6: return false;
7: });
There are several other helpful areas in my ASP.NET MVC application that I found jQuery to be very convenient, efficient, and resulted in a cleaner code base.
If you haven’t used jQuery before I highly recommend you check it out.
ASP.NET AJAX 4.0 CodePlex Preview 1
The future of ASP.NET AJAX can be found in the Roadmap document hosted on the CodePlex website.
The preview 1 release contains somewhat complete versions of the following features
- Client-side template rendering
- Declarative instantiation of behaviors and controls
- DataView control
- Markup extensions
- Bindings
Feel free to download the preview yourself and kick the tires.
.NET 3.5 Framework Poster
For those of you who like a visual representation of your commonly used types and namespaces, then you are in luck! Microsoft has conveniently created a nice poster highlighting Microsoft Framework 3.5.
Feel free to download the full copy from their website.
Design Pattern Riddle #6
But I'm just a thin outer case.
I typically call methods on another machine,
which might make bug tracking hard to trace.
a. Proxy
~/riddle by me
Friday, July 18, 2008
Design Pattern Riddle #5
I change my behavior depending on my mood.
You might think I am someone else,
But I’m just a class with an attitude!
a. State
~/riddle by me
Thursday, July 17, 2008
Design Pattern Riddle #4
And you need to switch from that to this.
Just abstract away those different pieces;
Then you’ll be living in encapsulated bliss.
a. Strategy
~/riddle by me
Wednesday, July 16, 2008
Design Pattern Riddle #3
I distribute it to those that subscribe
Some say I follow the Hollywood principle,
But I’ll tell you that c# delegates and I jibe.
a. Observer
~/riddle by me
Tuesday, July 15, 2008
Design Pattern Riddle #2
And your needs are dynamic and versatile,
Then I’m a good alternative to subclassing.
You will find that using me quite worthwhile.
a. Decorator
~/riddle by me
Tuesday, July 08, 2008
Design Pattern Riddle #1
I’m accessible to you just about everywhere.
I’m a solitary class, only one of me is made;
When you need me the most, I’ll come to your aid.
a. Singleton
~/riddle by me
Monday, July 07, 2008
Quality Grin
I am a good place to begin
You can find me in VS 2008;
All the green will make you grin.
a. MSTest
~/riddle by me
Thursday, July 03, 2008
Concise Description
But you might need to describe something twice.
I am most useful when it comes to LINQ;
You will find using me to be very concise.
a. Implicitly Typed Local Variables
~/riddle by me
Wednesday, July 02, 2008
Compiler Inferred
if you want to play with me.
I am inferred and created by the compiler;
before you know it I will flee.
Intellisense can pick me up and see me;
I am only readable inside.
I am useful when using LINQ;
where will you use me? You decide.
a. Anonymous Types
~/riddle by me
Tuesday, July 01, 2008
Complex Line
I can do it while you assign.
I am not an overloaded constructor;
You can write me in one line.
a. Object Initializer
~/riddle by me